Angular 19 HttpClient : HTTP POST Request
February 11, 2025
Angular provides HttpClient
to perform HTTP requests. To send HTTP POST request, use HttpClient.post
method. Find the steps to perform POST request. I am using Angular 19.
1. To use
HttpClient
, configuire provideHttpClient()
.
providers: [ provideHttpClient(), ]
HttpClient
using constructor.
@Injectable({ providedIn: 'root' }) export class WriterService { constructor(private http: HttpClient) { } }
HttpClient.post
method.
post(url: string, body: any | null, options: { headers?: HttpHeaders | { [header: string]: string | string[]; }; observe?: HttpObserve; params?: HttpParams | { [param: string]: string | string[]; }; reportProgress?: boolean; responseType?: 'arraybuffer' | 'blob' | 'json' | 'text'; withCredentials?: boolean; } = {}): Observable<any>
4.
createWriter(writer: Writer): Observable<Writer> { return this.http.post<Writer>(this.url, writer); }
1. ApplicationConfig
/app/app.config.tsimport { ApplicationConfig, importProvidersFrom } from '@angular/core'; import { provideHttpClient } from '@angular/common/http'; import { InMemoryWebApiModule } from 'angular-in-memory-web-api'; import { TestData } from './test-data'; export const APP_CONFIG: ApplicationConfig = { providers: [ provideHttpClient(), importProvidersFrom(InMemoryWebApiModule.forRoot(TestData, { delay: 1000 })) ] };
2. Create Service
/app/writer.service.tsimport { Injectable } from '@angular/core'; import { HttpClient, HttpHeaders, HttpResponse } from '@angular/common/http'; import { Observable } from 'rxjs'; import { Writer } from './writer'; @Injectable({ providedIn: 'root' }) export class WriterService { url = "/api/writers"; constructor(private http: HttpClient) { } createWriter(writer: Writer): Observable<Writer> { let httpHeaders = new HttpHeaders() .set('Content-Type', 'application/json'); let options = { headers: httpHeaders }; return this.http.post<Writer>(this.url, writer, options); } getAllWriter(): Observable<Writer[]> { return this.http.get<Writer[]>(this.url); } }
export interface Writer { id: number; name: string; city: string; }
3. Create Component
/app/writer.component.tsimport { Component, OnInit } from '@angular/core'; import { FormGroup, FormBuilder, Validators, ReactiveFormsModule } from '@angular/forms'; import { Observable, of } from 'rxjs'; import { WriterService } from './writer.service'; import { Writer } from './writer'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-writer', imports: [ReactiveFormsModule, CommonModule], templateUrl: './writer.component.html' }) export class WriterComponent implements OnInit { dataSaved = false; writerForm!: FormGroup; allWriters!: Observable<Writer[]>; constructor(private formBuilder: FormBuilder, private writerService: WriterService) { } ngOnInit() { this.writerForm = this.formBuilder.group({ id: ['', [Validators.required]], name: ['', [Validators.required]], city: ['', [Validators.required]] }); this.loadAllWriters(); } onFormSubmit() { this.dataSaved = false; let writer = this.writerForm.value; this.createWriter(writer); this.writerForm.reset(); } createWriter(writer: Writer) { this.writerService.createWriter(writer).subscribe({ next: writer => { console.log(writer); this.dataSaved = true; this.loadAllWriters(); }, error: err => { console.log(err); } }); } loadAllWriters() { this.allWriters = this.writerService.getAllWriter(); } get id() { return this.writerForm.get('id'); } get name() { return this.writerForm.get('name'); } get city() { return this.writerForm.get('city'); } }
4. Create HTML Template
/app/writer.component.html<h3>Create Writer</h3> @if(dataSaved && writerForm.pristine) { <p class="success"> Writer created successfully.</p> } <form [formGroup]="writerForm" (ngSubmit)="onFormSubmit()"> <table> <tr> <td>ID: </td> <td> <input formControlName="id"> @if(id?.dirty && id?.errors && id?.errors?.['required']) { <div class="error"> Id required. </div> } </td> </tr> <tr> <td>Name: </td> <td> <input formControlName="name"> @if(name?.dirty && name?.errors && name?.errors?.['required']) { <div class="error"> Name required. </div> } </td> </tr> <tr> <td>City: </td> <td> <input formControlName="city"> @if(city?.dirty && city?.errors && city?.errors?.['required']) { <div class="error"> City required. </div> } </td> </tr> <tr> <td colspan="2"> <button [disabled]="writerForm.invalid">Submit</button> </td> </tr> </table> </form> <h3>Writer Details</h3> @for(w of allWriters | async; track w) { <p> {{w.id}} | {{w.name}} | {{w.city}} </p> }
Test Data
/app/test-data.tsimport { InMemoryDbService } from 'angular-in-memory-web-api'; export class TestData implements InMemoryDbService { createDb() { let writerDetails = [ { id: '100', name: 'Mohit', city: 'PrayagRaj' }, ]; return { writers: writerDetails }; } }
6. Output
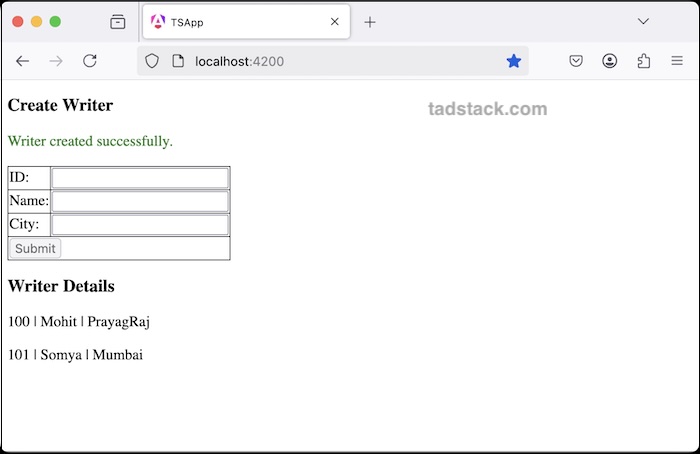