Using FormControl in Angular 19
February 12, 2025
Angular provides FormControl
to track value and status of an individual form control such as input, select, radio button, checkbox etc. Using FormControl
, we can get current value of form control and can set a default value and can change form control value at runtime. FormControl
can also apply validator on form control and we can track validation status. Validator can be added or removed at runtime. In this article, let us discuss using FormControl
step-by-step.
1. Create FormControl
ImportFormControl
from @angular/forms
.
import { FormControl } from '@angular/forms';
FormControl
as below.
customer = new FormControl();
customer = new FormControl("Mahesh");
formControl
as property binding.
A text will be created with value Mahesh.
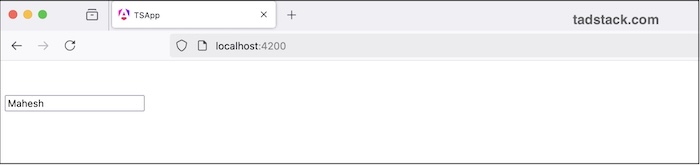
Call
value
property to get FormControl
current value.
console.log(this.customer.value);
2. Set and Patch Value
setValue : Sets a new value for the control.ngOnInit() { this.customer.setValue("David"); }
patchValue : Patches the value of a control.
this.customer.patchValue("David");
3. Create FormControl with Validators
To use validators withformControl
, pass it as second argument. For async validators, pass async validators as third argument. Here I am using only sync validators.
customer = new FormControl("", [Validators.required, Validators.minLength(3)]);
{{customer.status}}
FormControl
has boolean properties such as valid, invalid, pending, disabled, enabled, pristine, dirty, touched, untouched. We can use them in TS file as well as in HTML template.
4. Add and Remove Validators Dynamically
Add validators:const fnMinLength = Validators.maxLength(10); this.customer?.addValidators([fnMinLength]); this.customer?.updateValueAndValidity();
this.customer?.removeValidators([fnMinLength]); this.customer?.updateValueAndValidity();
5. Grouping FormControl
To groupFormControl
, use FormGroup
.
company.component.ts
import { Component, OnInit } from '@angular/core'; import { FormGroup, Validators, ReactiveFormsModule, FormControl } from '@angular/forms'; @Component({ selector: 'app-company', imports: [ReactiveFormsModule], templateUrl: './company.component.html' }) export class CompanyComponent implements OnInit { companayForm = new FormGroup({ id: new FormControl('', [Validators.required]), name: new FormControl(), location: new FormControl() }); onFormSubmit() { console.log(this.companayForm.value); } ngOnInit() { } }
company.component.html
<form [formGroup]="companayForm" (ngSubmit)="onFormSubmit()"> <p><input formControlName="id"></p> <p><input formControlName="name"></p> <p><input formControlName="location"></p> <p><button>Submit</button></p> </form>
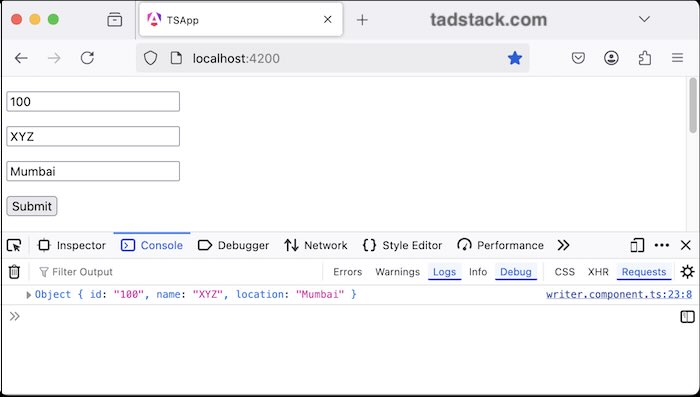