Using FormGroup in Angular 19
February 16, 2025
In this article, we will learn to use FormGroup
in our Angular 19 application.
1.
FormGroup
tracks the value and validity state of a group of FormControl
.
2. Create an instance of
FormGroup
as below.
customerForm = new FormGroup({ cid: new FormControl('', [Validators.required]), name: new FormControl(), });
FormGroup
that contains two FormControl
.
3. We bind the instance of
FormGroup
to HTML <form>
as below.
<form [formGroup]="customerForm" (ngSubmit)="onFormSubmit()"> <p>ID: <input formControlName="cid"></p> <p>Name: <input formControlName="name"></p> <p><button>Submit</button></p> </form>
FormGroup
instance with form, use formGroup
keyword.
To bind
FormControl
with form controls such as input text, use formControlName
keyword.
4.
FormGroup
and FormControl
can also be instantiated using FormBuilder
using its group()
and control()
method.
Find the code to create
FormControl
.
this.msg = this.formBuilder.control('Hello!');
FormGroup
.
this.customerForm = this.formBuilder.group({ cid: new FormControl('', [Validators.required]), name: new FormControl() });
1. Set Value
To set value, usesetValue()
method of FormGroup
. It overrides values of each form control within that FormGroup
. Suppose I have created FormGroup
as below.
customerForm = new FormGroup({ cid: new FormControl('', [Validators.required]), name: new FormControl(), city: new FormControl() });
setValue()
as below.
this.customerForm.setValue({ cid: '101', name: 'Jimmy', city: 'Mumbai' });
2. Patch Value
UsingpatchValue
, we can patch values to selected form controls within the FormGroup
. Suppose I have three form controls (cid, name, city) in FormGroup
and I want to update values only for two form controls (name, city) then we can use patchValue
.
this.customerForm.patchValue({ name: 'Mahesh', city: 'Varanasi' });
3. Reset
To resetFormGroup
, it provides reset()
method. It will reset values of all form controls.
this.customerForm.reset();
reset()
we can also set a default value to any form control.
this.customerForm.reset({ name: 'Bob' });
4. Complete Example
customer.component.tsimport { Component, OnInit } from '@angular/core'; import { FormGroup, Validators, ReactiveFormsModule, FormControl, FormBuilder } from '@angular/forms'; @Component({ selector: 'my-app', imports: [ReactiveFormsModule], templateUrl: './writer.component.html' }) export class CustomerComponent implements OnInit { constructor(private formBuilder: FormBuilder) { } customerForm = new FormGroup({ cid: new FormControl('', [Validators.required]), name: new FormControl(), city: new FormControl() }); onFormSubmit() { console.log(this.customerForm.value); this.customerForm.reset(); } ngOnInit() { } setValue() { this.customerForm.setValue({ cid: '101', name: 'Jimmy', city: 'Mumbai' }); } patchValue() { this.customerForm.patchValue({ name: 'Mahesh', city: 'Varanasi' }); } reset() { this.customerForm.reset({ name: 'Bob' }); } }
<form [formGroup]="customerForm" (ngSubmit)="onFormSubmit()"> <p>ID: <input formControlName="cid"></p> <p>Name: <input formControlName="name"></p> <p>City: <input formControlName="city"></p> <p><button>Submit</button></p> <p><button type="button" (click)="setValue()">Set Value</button></p> <p><button type="button" (click)="patchValue()">Patch Value</button></p> <p><button type="button" (click)="reset()">Reset</button></p> </form>
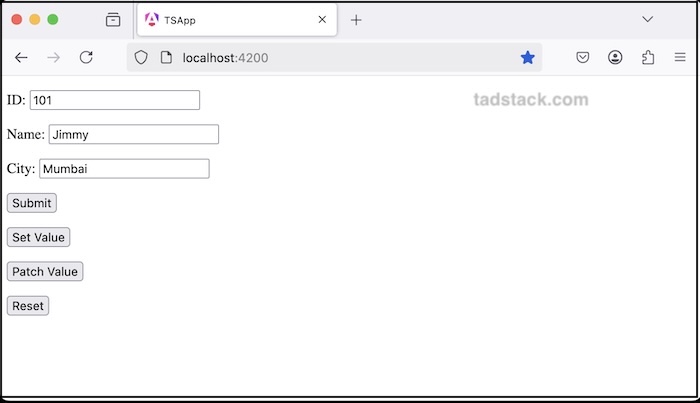